728x90
HttpURLConnection은 HTTP통신을 위한 클래스입니다. 이 클래스를 통해 웹페이지의 데이터를 받아오는 예제를 만들어 클래스의 기본적인 사용방법을 알아보도록 하겠습니다.
먼저 HTTP통신을 하기 위해 app -> manifests -> AndroidManifest.xml 파일에 다음과 같은 태그를 추가하여 인터넷 사용에 대한 권한을 명시합니다.
<uses-permission android:name="android.permission.INTERNET"></uses-permission>
MainActivity.kt의 onCreate() 메서드 안에서 HTTP를 요청할 URL 주소에 대한 객체를 생성합니다.
val url = URL("https://google.com/")
url객체에서 openConnection() 메서드를 호출하여 연결을 생성합니다. 이 메서드는 추상 클래스로서 이를 다시 HttpURLConnection이라는 구현 클래스로 형 변환하여 변수에 담아둡니다.
또한 requestMethod 속성을 통해 요청방식을 설정합니다. 요청은 GET, POST 등 대문자로 지정해야 합니다.
val huc = url.openConnection() as HttpURLConnection
huc.requestMethod = "GET"
연결 후에는 responseCode로 연결성공 여부를 판단합니다.
if (huc.responseCode == HttpURLConnection.HTTP_OK) {
}
성공적으로 연결되었으면 입력스트림을 생성하여 버퍼에 담아둡니다.
if (huc.responseCode == HttpURLConnection.HTTP_OK) {
val streamReader = InputStreamReader(huc.inputStream)
val buffered = BufferedReader(streamReader)
}
버퍼를 통해 읽어온 데이터를 한줄씩 문자열로 저장합니다.
if (huc.responseCode == HttpURLConnection.HTTP_OK) {
val streamReader = InputStreamReader(huc.inputStream)
val buffered = BufferedReader(streamReader)
val content = StringBuilder()
while (true) {
val data = buffered.readLine() ?: break
content.append(data)
}
}
데이터를 모두 읽었으면 버퍼와 연결을 모두 닫고 읽은 데이터를 Log를 통해 출력해 봅니다.
if (huc.responseCode == HttpURLConnection.HTTP_OK) {
val streamReader = InputStreamReader(huc.inputStream)
val buffered = BufferedReader(streamReader)
val content = StringBuilder()
while (true) {
val data = buffered.readLine() ?: break
content.append(data)
}
buffered.close()
huc.disconnect()
Log.d("결과값", "${content}")
}
마지막으로 이 전체의 동작을 모두 백그라운드에서 수행하도록 thread로 묶어 놓습니다.
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
thread(start = true) {
val url = URL("https://google.com/")
val huc = url.openConnection() as HttpURLConnection
huc.requestMethod = "GET"
if (huc.responseCode == HttpURLConnection.HTTP_OK) {
val streamReader = InputStreamReader(huc.inputStream)
val buffered = BufferedReader(streamReader)
val content = StringBuilder()
while (true) {
val data = buffered.readLine() ?: break
content.append(data)
}
buffered.close()
huc.disconnect()
Log.d("결과값", "${content}")
}
}
}
}
앱을 실행하고 결과를 확인합니다.
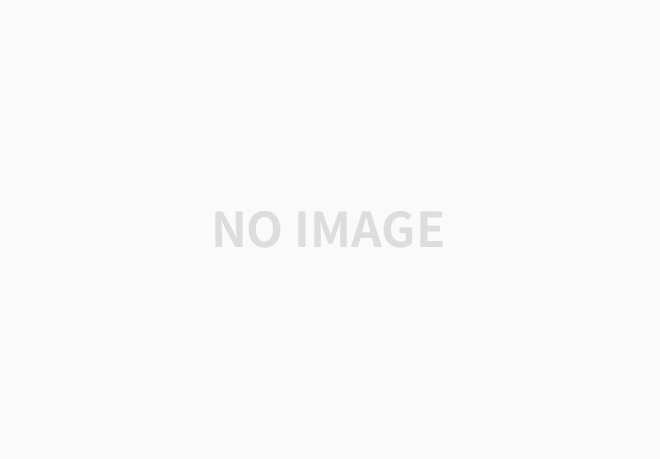
728x90
'Mobile > Kotlin' 카테고리의 다른 글
[Kotlin] 권한의 이해 (0) | 2021.02.24 |
---|---|
[Kotlin] 컨텐트 리졸버(Content Resolver) (0) | 2021.01.11 |
[Kotlin] 포어그라운드(Foreground) 서비스 (0) | 2021.01.08 |
[Kotlin] 서비스(Service) (2) | 2021.01.07 |
[Kotlin] AsyncTask (0) | 2021.01.06 |